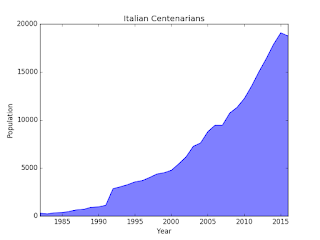
Data are coming from ISTAT, the Italian Institute for Statistics and shows how centenarian people boomed in Italy in the last decades.
The cool thing about it, especially if you are not Italian, is not much in the information you get but in the way I created the picture. Starting from the rough data, using python and the matplotlib library.
Here is the source code:
import matplotlib.pyplot as plt # 1 # 2 years = [ 1982, 1983, 1984, 1985, 1986, 1987, 1988, 1989, 1990, 1991, 1992, 1993, 1994, 1995, 1996, 1997, 1998, 1999, 2000, 2001, 2002, 2003, 2004, 2005, 2006, 2007, 2008, 2009, 2010, 2011, 2012, 2013, 2014, 2015, 2016 ] population = [ 302, 229, 345, 368, 482, 649, 703, 922, 960, 1120, 2852, 3053, 3273, 3562, 3690, 4015, 4377, 4516, 4765, 5435, 6153, 7265, 7614, 8797, 9470, 9470, 10728, 11319, 12243, 13544, 15029, 16390, 17884, 19095, 18765 ] plt.plot(years, population) # 3 plt.xlabel('Year') # 4 plt.xlim(years[0], years[-1]) # 5 plt.ylabel('Population') plt.title('Italian Centenarians') # 6 plt.fill_between(years, population, 0, color= 'blue', alpha= 0.5) # 7 plt.show() # 81. This is the standard way to import the pyplot sub-package. No one want to bring a long such long name, so the plt moniker is used instead.
2. If you don't believe me, you can get the data by yourself from ISTAT. Demography are from demo.istat.it.
3. I say to pyplot that I want to plot my data as a continuous line putting years on the X axis and population on Y.
4. Let the viewer better understand what's going on, showing a descriptive label.
5. Trim the picture so that it starts on the left with the first year and end to the right with the last one. Pyplot likes to let some room around.
6. It is always a good idea to give a name to the picture.
7. A nice touch, a bit of color to improve the readability.
8. After anything has been set on the area, it is time show it. This is the moment the image is actually generated.